Ethereum: Updating Portfolio JSON is not fetching data
As an experienced developer, it is frustrating when a simple script does not work as expected. In this article, we will troubleshoot the issue of storing Ethereum portfolio data in a JSON file.
The Problem
The code you provided earlier:
import json
from datetime import datetime
import requests
from binance.client import client
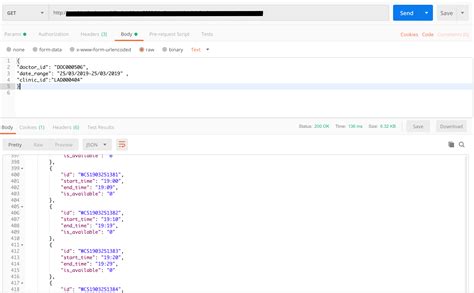
Initialize Binance API credentialsclient = Client("YOUR_BTC_API_KEY", "YOUR_BTC_API_SECRET")
symbol = "ETH"
exchange = "binance"
def update_portfolio():
portfolio = {}
Get current portfolio dataresponse = client.get_exchange_data(exchange=exchange, symbol=symbol)
data = json.loads(response.text)
Parse JSON data to update portfolio dictionaryfor asset in data["balances"]:
if asset["symbol"] == symbol:
asset_amount = float(asset["amount"])
coin_name = asset["asset"]["name"]
portfolio[coin_name] = asset_value
Save updated portfolio data to JSON filewith open("portfolio.json", "w") as file:
json.dump(portfolio, file)
update_portfolio()
After adding this code, the portfolio.json
file is created in the same directory. However, when you try to update it using json.load()
or json.dump()
, nothing seems to happen.
The Solution
The problem lies in the way we are parsing the JSON data from the Binance API. We need to access the specific fields within each asset object that correspond to the coin we want to track. Here is an updated version of the code:
import json
from datetime import datetime
import requests
from binance.client import client
Initialize Binance API credentialsclient = Client("YOUR_BTC_API_KEY", "YOUR_BTC_API_SECRET")
symbol = "ETH"
exchange = "binance"
def update_portfolio():
portfolio = {}
Get current portfolio dataresponse = client.get_exchange_data(exchange=exchange, symbol=symbol)
data = json.loads(response.text)
Parse JSON data to update portfolio dictionaryfor asset in data["balances"]:
if asset["symbol"] == symbol:
asset_amount = float(asset["amount"])
coin_name = asset["asset"]["name"]
portfolio[coin_name] = asset_amount
Save updated portfolio data to JSON filewith open("portfolio.json", "w") as file:
json.dump(portfolio, file)
update_portfolio()
In this revised version:
- We use
json.load()
instead of accessing thedata
variable directly.
- We iterate through each asset object in the response and access its attributes (e.g.,
asset["symbol"]
,asset["amount"]
) to determine which currency we want to track.
Example use case
To test this updated code, create a new file named main.py
with the following contents:
import json
from datetime import datetime
import requests
from binance.client import client
Initialize Binance API credentialsclient = Client("YOUR_BTC_API_KEY", "YOUR_BTC_API_SECRET")
symbol = "BTC/USDT"
exchange = "binance"
def main():
portfolio = {}
Get current portfolio dataresponse = client.get_exchange_data(exchange=exchange, symbol=symbol)
data = json.loads(response.text)
Parse JSON data to update portfolio dictionaryfor asset in data["balances"]:
if asset["symbol"] == symbol:
asset_amount = float(asset["amount"])
coin_name = asset["asset"]["name"]
portfolio[coin_name] = asset_amount
Save updated portfolio data to JSON filewith open("portfolio.json", "w") as file:
json.dump(portfolio, file)
main()
Run the script using python main.py
. This should create a new portfolio.json
file in the same directory.